Spring Framework is a popular framework that simplifies the development of Java enterprise applications by providing infrastructure support and dependency management. This makes it easier for developers to stay focused on solving business problems instead of technical and configurational challenges.
Spring framework history
In October 2002, Rod Johnson published a book called “Expert One-on-One J2EE Design and Development”. In his book, Johnson proposed new approaches based on plain Java objects (POJOs) for the development of Java enterprise applications. His ideas proposed in the ebook helped eliminate deficiencies and extend the J2EE platform’s popularity in those days. Also, Johnson shared an example application and its sources, using the new approaches contained in the book.
In a matter of months, developers from across different industries adopted Rod Johnson’s approaches. The consequences were quick to make themselves evident and sure enough, software engineers Juergen Hoeller and Yann Caroff convinced Johnson to develop an open source project based on the infrastructure code of the example application provided in his book. As a result, in February 2003, all three of them began working on a project they decided to name “Spring”.
“Whatever happened next, the framework needed a name. In the book, it was referred to as the “Interface21 framework” (at that point it used com.interface21 package names), but that was not a name to inspire a community. Fortunately, Yann stepped up with a suggestion: “Spring”. His reasoning was associated with nature (having noticed that I'd trekked to Everest Base Camp in 2000); and the fact that Spring represented a fresh start after the “winter” of traditional J2EE. We recognized the simplicity and elegance of this name, and quickly agreed on it” - as Rod Johnson writes
The first Spring release under the Apache 2.0 license was made available in June 2003. The production version was released in March 2004.
Comparing Spring with non-Spring infrastructure
When comparing Spring with non-Spring infrastructure, it always implies Java EE (Jakarta EE), because many will find that the Java Standard Edition doesn’t necessarily have comparable classes and features with Spring. For example, if you try to write the same app on Java SE and Spring separately, you will find yourself with a ton of boilerplate code needed to write if using Java SE only. In that scenario, it should always be taken into account that most applications written on both technologies are web so most infrastructure built on top of each is web app-oriented.
Table 1. Comparison Spring vs Java EE
Spring Framework | Java EE (Jakarta EE) |
Advantages The same principles | Context Dependency Injection: The same principles |
Spring Beans: Simple POJOs | Enterprise Java Beans: Different types of web-oriented features that are not always needed |
Popular web server support | Limited web server support or integrated server |
Apache 2.0 open-source license | Eclipse/Oracle paid license |
Big community | Eclipse/Oracle support |
Less time to develop | More time to develop |
High adoption rate | Low adoption rate |
Less expensive | More expensive |
Kotlin, Groovy support | Java only |
Latest versions of Java SE | Java 11 SE is the latest |
Lower performance | Higher performance |
Though Jakarta EE has a high performance, it still has a lower quantity of features and support when compared to Spring.
Main Spring projects
Spring Boot is Spring’s most popular project and for good reason. This project makes it easy to start and run applications with automatic configuration, giving you a large set of starter artifacts that include all needed dependencies out-of-the-box. It’s also embedded with web servers, which is a big plus. On the downside, the Spring Boot project comes with a big number of unused dependencies, which doesn’t fit well when it comes to building monolithic applications.
Spring Data project makes it easy to work with databases, it eliminates unnecessary boilerplate JDBC-connection-statements code. One of its drawbacks is slower performance when compared to basic JDBC but not as significant for web applications.
Spring MVC project implements the Model-view-Controller pattern that is widely used for web application development. This project is built on the Servlet API and provides interfaces for web servers using the request-response model.
Spring WebFlux project offers support for the Reactive Streams API.
Spring Session project provides an API and implementation for managing a user’s session information.
Spring Security project handles authentication and authorization, and it easily integrates with Spring MVC and the Servlet API.
Spring fundamentals overview
The Spring Framework is based on three core concepts:
- Inversion of Control (IoC)
- Spring Bean
- Dependency Injection (DI)
Next, we’re going to break down each of these core concepts in greater detail.
Inversion of Control (IoC) is a programming principle that ensures the control flow of a program is inverted from how it is usually done where custom-written code is used to call libraries to solve specific tasks. In IoC, we hand over control of the program to the framework and just add our own parts to the main framework's control flow. The IoC principle was put into practice by the Spring IoC container in the Spring Framework.
Figure 1. The Spring IoC container
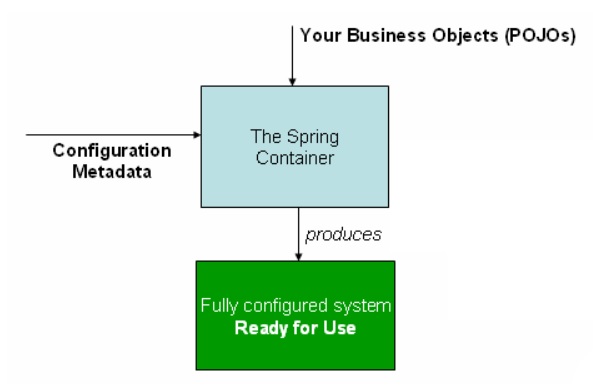
Next up, we’re going to dive a little deeper into Spring Bean, an important term that describes the basic framework’s units on which the whole system is built.
You can find the exact Spring Bean definition in the official Spring Framework documentation, which states the following:
“In Spring, the objects that form the backbone of your application and that are managed by the Spring IoC container are called beans. A bean is an object that is instantiated, assembled, and managed by a Spring IoC container. Otherwise, a bean is simply one of many objects in your application. Beans, and the dependencies among them, are reflected in the configuration metadata used by a container.
The interface org.springframework.context.ApplicationContext represents the Spring IoC container and is responsible for instantiating, configuring, and assembling the aforementioned beans. The container gets its instructions on what objects to instantiate, configure, and assemble by reading configuration metadata. The configuration metadata is represented in XML, Java annotations, or Java code. It allows you to express the objects that compose your application and the rich interdependencies between such objects.”
To learn more about how to create configuration metadata and run applications based on the Spring Framework, we recommend you take a look at the Spring documentation which comes in very handy.
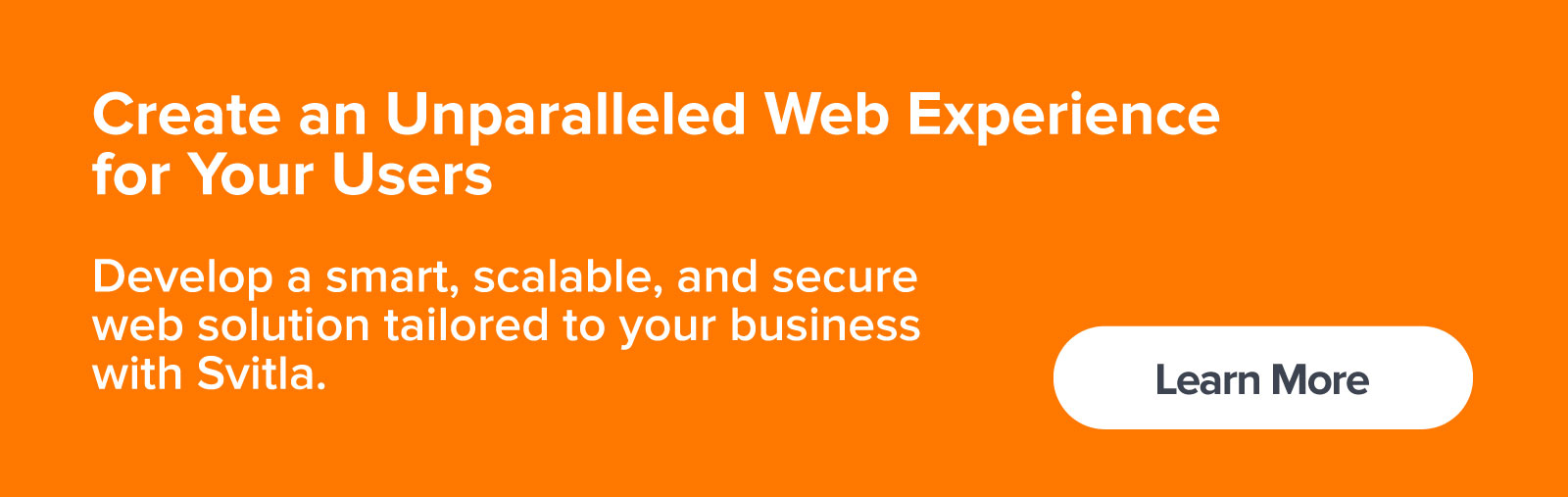
Now, we couldn’t talk about the Spring Framework and not mention Dependency Injection (DI). Dependency Injection is the process where beans from configuration metadata are automatically injected in places where they are needed and called.
Next, we’ll be showing you the three types of dependency injection.
Constructor-based DI:
public class SimpleMovieLister {
// the SimpleMovieLister has a dependency on a MovieFinder
private final MovieFinder movieFinder;
// a constructor so that the Spring container can inject a MovieFinder
public SimpleMovieLister(MovieFinder movieFinder) {
this.movieFinder = movieFinder;
}
// business logic that actually uses the injected MovieFinder is omitted...
}
Code language: PHP (php)
Listing 1. Constructor-based DI example
You don’t need to manually call an implementation constructor as the IoC container will take this task over.
Setter-based DI:
public class SimpleMovieLister {
// the SimpleMovieLister has a dependency on the MovieFinder
private MovieFinder movieFinder;
// a setter method so that the Spring container can inject a MovieFinder
public void setMovieFinder(MovieFinder movieFinder) {
this.movieFinder = movieFinder;
}
// business logic that actually uses the injected MovieFinder is omitted...
}
Code language: PHP (php)
Listing 2. Setter-based DI example
Field-based DI using @Autowired annotation:
public class SimpleMovieLister {
// the SimpleMovieLister has a dependency on the MovieFinder
// an @Autowired annotation so that the Spring container can inject a MovieFinder automatically
@Autowired
private MovieFinder movieFinder;
// business logic that actually uses the injected MovieFinder is omitted...
}
Code language: PHP (php)
Listing 3. Field-based DI example
As you can see, all Spring infrastructure is based on these three core concepts and everything else simply features that solve specific programming problems like a web application, database connections, cache, security, testing, configuration formats, and more.
Spring testing support
Aside from the clear project infrastructure embodied by Inversion of Control and Dependency Injection, the Spring framework also provides you with a well-configured testing environment, which is an added bonus.
Spring's ability to handle a system as a set of simple POJOs gives you the opportunity to effectively use SOLID principles and make code less dependent on the container than it would be with traditional Java EE development, which in turn results in modularity and testability by common Java test frameworks such as JUnit or TestNG.
Spring’s testing support packages consist of classes dedicated to its project feature and infrastructure integration testing.
Project-feature testing support includes Spring MVC, Servlet API, JDBC, Spring Reactive and Transaction management testing classes. Testing infrastructure support includes the ability to cache Spring IoC container among tests, reducing the time needed to run each test together, and providing Dependency Injection of test fixture instances.
In addition, Spring has a number of mocking and utility classes and annotations for use in unit and integration testing. These elements help solve several problems including reflection access to inaccessible fields, dependency injection in tests and tested classes, Mockito mocks injection, AOP support, and more.
The Spring team promotes and contributes to the use of Test-driven development (TDD). These intentions can be observed implemented in the Spring testing features.
What does the future hold for Spring?
Since its initial release in 2003, the Spring framework has surely come a long way yet it still caters to the foundational need of making Java server-side development easier, allowing development teams to more quickly create applications. Which in a way, is what every developer dreams of.
Now, the Spring framework is a household name that continues to grow with no signs of stopping. Developers see no need to replace their Spring infrastructure with anything else given the lack of potential competitors and the high cost it would represent to implement a replacement.
As of today, one promising vector in Spring is the Reactive stack, so we can expect that in the near future, we will see new features for extending WebFlux.
In summary, we can confidently say that Spring is certainly worth your time, attention, and resources, and we highly recommend that you employ developers who are well-versed in the nuances of Spring to speed up your development endeavors.
At Svitla Systems, we’ve worked on a rich variety of Spring-based projects with our skilled professionals who help bridge the gap between your requirements and your end goals, using the best, most cutting-edge technologies that benefit your project.
Care to learn more? Reach out to our representatives so we can give you more details of how we can help you.