Since the number of services that actively use REST protocol, including cloud systems and microservices, has recently increased, the question of high-quality and quick testing of REST-based APIs becomes even more relevant. Manually reviewing each request and generating parameters is a rather laborious task, as when changing the back-end or the microservice architecture settings, you need to check the API constantly during the development process. This is where the REST API testing tools are used.
Table of contents:
- What is REST?
- Why test REST API?
- The methodology of testing REST.
- Curl for testing API.
- Postman for testing API.
- Conclusion.
What is REST?
REST (Representational State Transfer) provides communication between the client (usually a browser) and the server using ordinary HTTP requests (GET, POST, PUT, DELETE, etc.). It transfers information from the client in the request parameters, information from a server in the body of the response (which can be, for example, a JSON object or an XML document).
REST is a software architectural style for web services. Actually, it is not a protocol itself, but a set of rules or guidelines for building an effective communication model between the sender and receiver of information. RESTful Web services are built with a list of constraints that simplify client-server information exchange and their software implementation.
REST API works over HTTP protocol. RESTful service uses four methods from HTTP protocol: get, post, put, and delete. These methods are restricted to storing client status on the state server, i.e. “stateless”.
In turn, HTTP (HyperText Transfer Protocol) is an application-level data transfer protocol that was originally planned in the form of hypertext documents in the HTML format and is currently used to transfer arbitrary data. The basis of HTTP is the client-server technology, that is, the following are assumed:
- Consumers (customers) who initiate a connection and send a request;
- Providers (servers) who are waiting for a connection to receive a request, take the necessary actions, and return a message with the result.
The RESTful application builds logic around resources, but not around endpoints:
- Architectural style with no standardized description.
- Stateless (no server-side information about client state).
- A cache is available for API calls.
- One presentation layer works with JSON, XML, HTML, etc.
- Works well on the public API level, microservices, and systems with high scalability.
Why test REST API?
The task of testing the API helps to clearly determine in which part the error occurs. Error localization at the separation level of the server and client parts allows you to quickly find the place with the wrong logic of the information system. And testing the REST API lends itself to mechanization and can be effectively performed by tools such as curl and Postman.
The ability to work with the API allows you to better understand and more accurately describe the errors that have occurred. Suppose you open a certain page of a site and see an error message in the user interface. At the API level, tracking down such errors is much easier and faster than trying to look at the code and figure out the location with the wrong functionality.
There are projects where some of the tests will have to be carried out only through program interfaces. The architecture of microservices is gaining more and more popularity when the application is not "monolithic", but in fact, is a set of stand-alone applications with data exchange. In this case, API testing becomes especially popular. It is this that allows you to fully verify the correct integration of individual services and observe the logic of the functioning of the system as a whole.
The methodology of testing REST
How to test Rest API? The concept of testing is based on sending test HTTP requests to the URL with mock data. It allows us to compare received answers from API servers and compare it with expected data. Let's take a look at the process of testing Rest API.
The testing methodology of the REST API is quite simple and intuitive in practice. To do this, select and install one of the means of generating REST requests. There are a lot of them. Mostly, in practice, curl and Postman are used. The logic of testing the REST protocol looks like this in general:
- form data for the request
- form a command for the request and select the request method (GET, POST, PUT, DELETE)
- pass the command to curl (or to Postman)
- get a response from the server (in the form of HTTP code, and data, for example in JSON, HTML, XML) and write to a file
- compare the results from the server and the expected value (you can use the diff utility)
The general diagram of the testing methodology can be seen below in the figure.
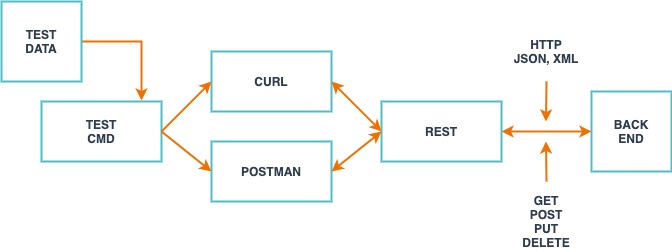
The main types of API testing are listed on this page and are as follows:
- Unit testing - Testing the functionality of individual operations.
- Functional testing - Testing the functionality of broader scenarios, often using unit tests as building blocks for end-to-end tests. Includes test case definition, execution, validation, and regression testing.
- Load testing - Validating functionality and performance under load, often by reusing functional test cases. For example, the Postman load test allows the user to perform complex testing of given servers. Please refer to more information in this article.
- Runtime error detection - Monitoring an application the execution of automated or manual tests to expose problems such as race conditions, exceptions, and resource leaks.
Let's compare the two most popular tools for testing Rest API: curl vs. Postman. These tools are perfect for the given task, and each has advantages. Let's start with the console tools curl and then compare it to the GUI-powered tool Postman. And in some cases, testing API requires using both curl and Postman.
Curl for testing API
Сurl is a cross-platform command-line utility that allows you to interact with various servers using a variety of different protocols with URL syntax. cURL can automate file transfers or a sequence of such operations. For example, it is a good tool for modeling client actions with an API.
Let's take a quick look at testing REST APIs using curl. Curl is a command-line tool for transferring data and supports about 22 protocols, including HTTP. This combination makes it a very good special tool for testing almost any REST service.
Command-line options
Curl supports over 200 command-line options. You can run a REST request either without parameters or with one or more parameters to accompany the URL request in the command. Let's look at two simple parameters that would facilitate the testing process.
Verbose mode
When testing more complex queries, we recommend setting the verbose mode to -v:
curl -v http://example.com/
* Trying 93.184.216.34...
* TCP_NODELAY set
* Connected to example.com (93.184.216.34) port 80 (#0)
> GET / HTTP/1.1
> Host: example.com
> User-Agent: curl/7.54.0
> Accept: */*
>
< HTTP/1.1 200 OK
… (HTML)...
Code language: JavaScript (javascript)
As a result, the teams will provide useful information, such as the IP address, the port to which you are connecting, and headers.
Output
By default, curl prints the response body to standard output. If necessary, you can provide an output option to save to a file:
curl -o out.json http://www.example.com/index.html
Code language: JavaScript (javascript)
This is especially helpful when the response size from the REST request is large enough. You can examine in detail all the necessary data from the server response.
Each HTTP request contains a request method. The most commonly used methods are GET, POST, PUT and DELETE.
GET Method
This is the default method when making HTTP calls using curl. In fact, the previously shown examples were simple GET calls. When starting a local service instance on port 8080, you can use this command to call GET:
curl -v http://localhost:8082/
Code language: JavaScript (javascript)
POST method
This method is used to send data to the received service. And for this, we use the data option. The easiest way to do this is by putting data in a command:
curl -d 'id=1&name=create' http://localhost:8080/new
Code language: JavaScript (javascript)
or you can transfer the file containing the request body to the data option as follows:
curl -d @request.json -H "Content-Type: application/json" http://localhost:8080/new
Code language: CSS (css)
PUT Method
This method is very similar to POST. But it is used when you need to send a new version of an existing resource. To do this, use the -X option. Without any mention of the type of the request method, curl uses GET by default. Therefore, we explicitly mention the type of method in the case of PUT:
curl -d @request.json -H 'Content-Type: application/json' -X PUT http://localhost:8080/update
Code language: CSS (css)
DELETE Method
Again, we indicate that we want to use DELETE using the -X option:
curl -X DELETE http://localhost:8082/
Code language: JavaScript (javascript)
The main disadvantage and at the same time the main advantage of curl is the lack of a GUI.
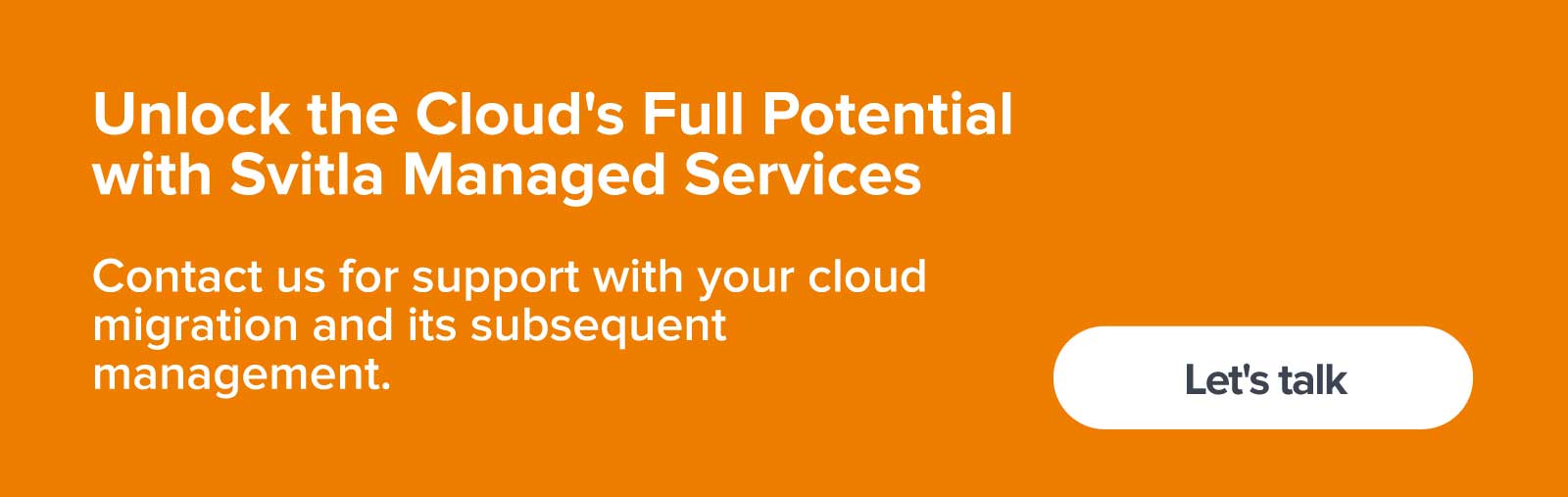
Postman for testing API
Postman is designed to check requests from the client to the server and receive a response from the back-end with a great user interface. It is also known as Postman REST Client. The developers of this tool say “Postman is a powerful set of API testing tools that have become a must for many developers. We make great products to help you create stunning APIs and improve development productivity. Postman is used by more than a million developers around the world, and this number is constantly growing. We plan to develop other products to provide developers with the most powerful solution for developing and testing APIs." Postman provides a graphical user interface for generating and validating requests.
Postman-level automation allows you to perform the following test steps:
- Saving Tests
- Create test collections
- Postman Standard Tests
- Modify tests for yourself in the old and new version
- Get data from object tree JSON, XML
- Manage environment variables
- Run one test several times
- Download test data from file
- Create a lot of data with unique values
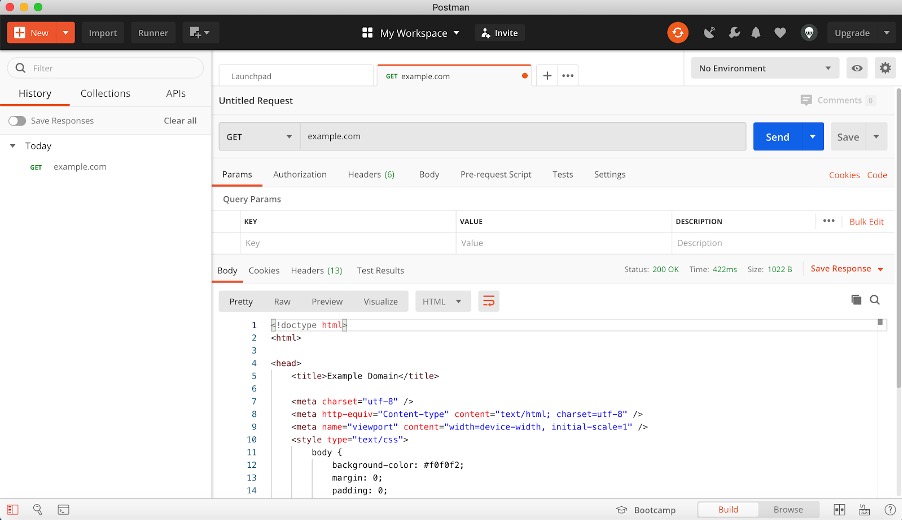
It is also very easy and understandable to use the menu to select the type of request.
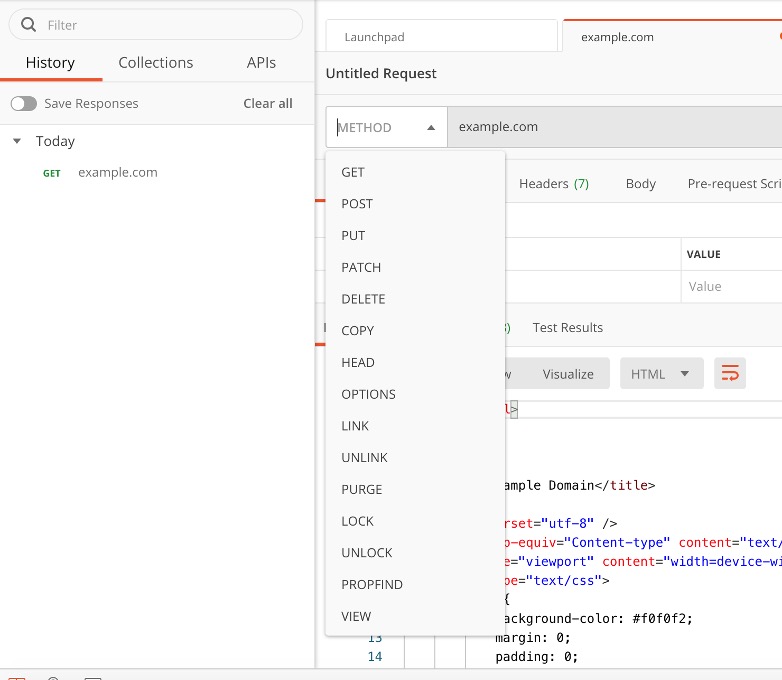
And what is very important, Postman has the ability to write a testing script. JavaScript is used for this. The presence of JavaScript allows many developers to easily join the testing process and write small, but effective test scripts. On the Postman documentation site, there are very clear and varied examples of writing test scripts, it is highly recommended that you look at them and automate the verification of the most common requests to test the API in your project.
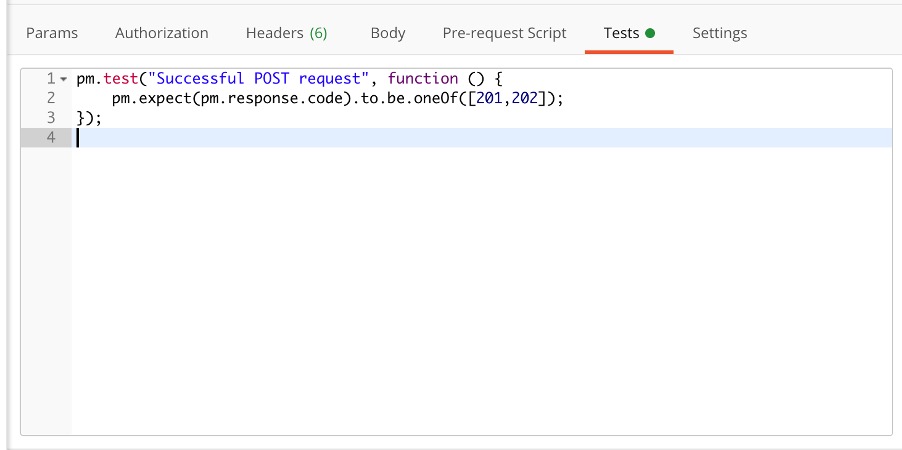
Postman (and curl) now implements all the necessary tools for testing complex structures in the REST API, and any service that works with HTTP requests can be fully tested with these tools. Regardless of the complex authorization mechanism, no matter how request parameters are formed, and whatever the format of the requested output, these two tools will cope with the task. The popularity and accessibility of these tools make working with them easy in view of the presence of a huge number of examples.
Conclusion
It is important in the current conditions of the development of microservices, the active use of APIs, cloud technologies to correctly build development processes, especially with regard to testing. Tools such as curl and Postman are essential and effective tools for supporting such development processes. We made a brief and comprehensive comparison of Postman vs. curl in this article.
In conclusion, it is worth noting that:
- Curl and Postman work on all platforms, including Windows, Linux, MacOS
- Both test tools are free.
- There is no ideal testing tool, for certain projects a certain tool is better
- You can automate the API testing process in both curl and Postman
- You can build a system of complex queries, including authorization, parameterization, support for various formats
It is very important to maintain the integrity of the API. In Svitla Systems we always recommend test methods and tools for testing REST API. As a result, lots of time and resources are saved when debugging projects and introducing new features.