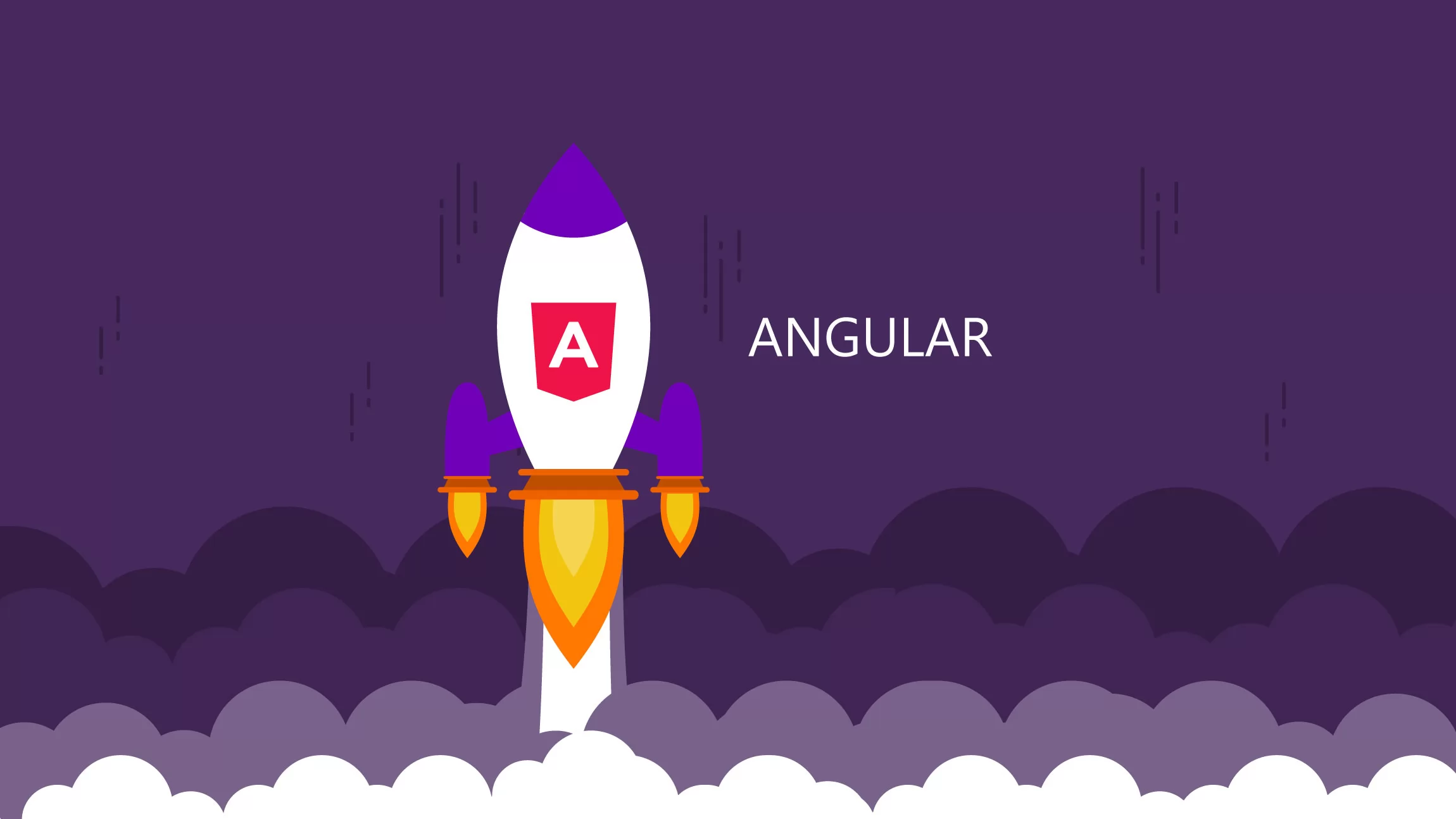
Angular
Any web project in the modern IT world needs a high-quality and convenient user interface. Web projects use HTML/CSS and JavaScript technology on the browser side to provide the user with a fast and efficient user interface. To build these kinds of web interfaces, you need to have a high-quality toolkit for working with UI controls and a convenient programming environment. For many years, developers around the world have been looking for the most logical and architecturally correct solution for building a web UI using JavaScript and Typescript.
The Angular framework is one of the most common and convenient front-end development tools. It’s intensively popularized by Google in a wide circle of both novice top IT executives and senior developers with extensive experience. Angular is based on the concept of a single-page web application. The application is built as a single HTML page based on the Model-View-Controller (MVC) pattern. This structure allows you to quickly develop and easily test the front-end part of the web-based information system. Angular is a frontend part of the MEAN stack, consisting of the MongoDB database, the Express.js web application development framework, Angular itself, and the Node.js platform. Also, NestJS is a framework built with NodeJS that allows the Angular app to make server-side rendering and generating static websites.
Angular Conception
Angular is a development platform that uses TypeScript. The Angular platform includes the following parts:
- A component-based framework for building scalable web applications.
- A collection of well-integrated libraries that cover a wide variety of features, including routing, forms management, client-server communication, and more.
- A suite of developer tools to help develop, build, test, and update your code.
Angular is designed with the belief that declarative programming is best suited for building user interfaces and describing software components, while imperative programming is best suited for describing business logic. A great idea underlies the framework, namely the separation of DOM manipulation and program logic. This division simplifies code testing. The complexity of testing largely depends on the structure of the code. The framework adapts and extends traditional HTML to provide two-way data binding for dynamic content, allowing automatic synchronization of model and view. As a result, Angular reduces the role of DOM manipulation to increase performance and simplify testing.
In Angular applications, components are the main building block. Every component has:
- An HTML template that declares what is rendered on the page.
- A Typescript class that defines the behavior.
- A CSS selector that defines how the component is used in a template.
- CSS styles applied to the template (optionally). It is possible to use less and sass as well.
Angular uses templates for rendering web pages in the browsers. As mentioned in the Angular documentation: “Each Angular template in your application is a section of HTML that you can include as a part of the page that the browser displays. An Angular HTML template renders a view, or user interface, in the browser, just like regular HTML, but with a lot more functionality.” Templates allow you to operate with interpolation, statements, binding, reference variables for DOM elements, events, SVG, etc. Also, Angular supports built-in directives. Directives are classes that add additional behavior to elements in your Angular applications. With Angular's built-in directives, the application manages forms, lists, styles, and all the elements of the graphical user interface.
Additionally, Angular can use attribute directives. With attribute directives, you can change the appearance or behavior of DOM elements and Angular components. Dependency Injection (DI) is a widespread design pattern. In Angular, DI allows you to create an object using other objects. At the same time, changes in the definition of the used objects do not affect the created object in any way. The core of Angular has its own implementation of the Dependency Injection pattern. Without it, it would be simply impossible to create an application. The simplest example of DI in Angular is when a component uses a service most often to retrieve data.
In order for the created service to be used by a component or other service, its declaration must be preceded by the @Injectable () decorator. Since services are created specifically for third-party use, it is recommended to always use a decorator. All services are registered by the Injector, which is part of the DI engine in Angular. Moreover, the application can have several injectors at the same time.
Angular Router is a powerful JavaScript router created on the Angular platform. It can be installed from the @angular/router package. You get a routing library with which you can define several router outlet elements, several strategies for matching the route to the query string, easily obtain route parameters and use hooks for authorized access to components. Angular Router is the foundation of the Angular platform. The router allows you to create one-page applications with multiple views and navigate them.
Angular pipe (well, actually, it's just a filter) is needed to transform data directly in an HTML template. For example, displaying the date and time in the format you want, or specifying the output format for a numeric value. Angular has a number of built-in filters, but you can also create your own.
Angular allows you to add validation to a form based on a template. In doing so, the developer adds the same validation attributes as with inline HTML form validation. Angular uses directives to map these attributes to the validator functions in the framework. You can also use Angular reactive forms, which are built on the basis of a mechanism that uses a reactive approach to programming. To do this, you need to import the ReactiveFormsModule. Interestingly, the very process of creating and validating Angular reactive forms is carried out directly in the controller.
Angular starts the check and generates a list of errors (these will be flagged with the INVALID status), or null (these will be flagged with the VALID status). Angular also supports input validation in reactive forms. Additionally, validator functions can be synchronous or asynchronous. The coding style guide is used to format the source codes of the project. This allows all developers to write equally high-quality code and adhere to the same style of design. More details can be found in the coding style guide here.
The uniqueness of Angular as a front-end web writing framework.
In fact, Angular is a unique framework for creating a front-end for modern web projects. It has a clear and simple architecture, modular and component structure, and allows you to solve modern problems on the development of effective user interfaces for web platforms and for mobile systems. With several valuable features, let’s talk about Angular’s most prominent ones.
Registration of callbacks: Registration of callback functions litters the code. It is always good to delete template code (such as callback functions). This significantly reduces the amount of code you need to write and improves the reading and comprehension of application code written in Angular.
Software DOM manipulation: Angular allows you to declaratively describe how the UI should change depending on the change in the state of the program. This frees you from low-level manipulation of the DOM. Most applications written with Angular never require DOM software manipulation, although this can be done if necessary.
Data flow from/to UI: Most operations in AJAX applications are CRUD operations. There is a data stream from the server to the internal object and then to the HTML form. This allows users to change the form, check the correctness of the data, and show validation errors. And only then, returning to the internal model and the server, which creates too much template code. Angular gets rid of most of this code, but it is allowed to leave the code describing the general data flow in the application, instead of details of implementation.
No large amount of code to write: You usually need to write a lot of extra code to get a simple "Hello World" AJAX application. With Angular, you can quickly create an application using services. These services are automatically embedded in the application in a Guice-like dependency-injection style. This allows you to quickly start developing the application. And you get full control over initialization in automated tests.
Some effectiveness of Angular is based on the AOT compiler in most cases. As mentioned on the Angular website: “An Angular application consists mainly of components and their HTML templates. Because the components and templates provided by Angular cannot be understood by the browser directly, Angular applications require a compilation process before they can run in a browser. The Angular ahead-of-time (AOT) compiler converts your Angular HTML and TypeScript code into efficient JavaScript code during the build phase before the browser downloads and runs that code. Compiling your application during the build process provides a faster rendering in the browser.”
Let’s mention some advantages of using AOT in Angular:
- For fast rendering with AOT, the browser loads the precompiled executable code of the application without first compiling the application.
- Less asynchronous ajax requests. The compiler embeds external HTML templates and CSS stylesheets into the JavaScript application.
- Provides a smaller downloadable Angular framework. There is no need to download the Angular compiler as the application is already compiled, which improves the payload of the application.
- Template errors are identified during the build phase before users can see them.
- Enhanced security. The AOT compiles HTML templates and components into JavaScript files before being passed to the client. This reduces the potential for injection attacks.
Also, Angular uses a next-generation compilation and rendering pipeline named Ivy. In modern versions of Angular, the View Engine is a new compiler and runtime instructions are used by default: ”AOT compilation with Ivy is faster and should be used by default. In the angular.json workspace configuration file, set the default build options for your project to always use AOT compilation. When using application internationalization (i18n) with Ivy, translation merging also requires the use of AOT compilation.“ Also in Angular, you can use the NgModule widget to create a component, directive, or channel. They will be available to external NgModules. This will allow you to import the widget into any NgModules. Many third-party UI component libraries are represented as a NgModules widget. The NgModule widget must be composed entirely of declarations. Most of these declarations will be exported. A widget rarely needs providers.
An example of building a simple application on Angular
There are many methods for building an Angular application. One of them is using the CLI. Let's take a look at what is needed for this. First, go to https://angular.io/cli. There, you’ll find instructions on how to install Angular CLI:
npm install -g @angular/cli
ng new my-first-project
cd my-first-project
ng serve
Now, let's take a look at the page of the application that was created using this template. Next, we can modify the files of this application to build our project. Go to the browser: http://localhost:4200/
Next, let's add Material Design components to our project. For example, we will use the button and when we click on it, we will show the snack-bar window. To do this, run the following command: ng add @angular/material
Next, write the necessary lines for the button and snack bar to the files.
File: app.module.ts
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { MatButtonModule } from '@angular/material/button';
import { MatSnackBarModule } from '@angular/material/snack-bar';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
AppRoutingModule,
BrowserAnimationsModule,
MatButtonModule,
MatSnackBarModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {
}
File: app.component.html
...
<h1>Angular Button</h1>
<div>
<button mat-raised-button color="primary" (click)="clicked()" >Welcome</button>
</div>
...
File: app.component.ts
import { Component } from '@angular/core';
import { Router } from '@angular/router';
import { MatSnackBar } from "@angular/material/snack-bar";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private snackBar: MatSnackBar) {}
title = 'my-first-project';
clicked() {
this.snackBar.open('Welcome to Angular Development');
}
}
Note that the clicked() method in the AppComponent class was written to handle the response to clicking the button. Once launched, you’ll get the new button displayed at the bottom of the window.
After clicking on the “Welcome” button, you will get the next snack bar window.
Unit Testing
To make sure your Angular application works correctly, you need to continuously test it. The application code should be tested regularly, and this process should be automated. It is necessary to prepare test scripts and start running them in the development cycle. There are two main types of testing the client-side of a web application program:
- Unit testing, which validates that small blocks of code accept expected inputs and return expected results. This type of testing deals with checking isolated parts of the code, mainly open interfaces.
- End-to-end testing, proving the performance of the entire application in line with the expectations of end-users. For end-to-end testing of Angular applications, it is recommended to use the Protractor library.
Jasmine is a very popular behavior-driven testing framework for JavaScript. It allows you to test front-end and Node.js, and doesn’t need external dependencies. Since most developers are already accustomed to the Jasmine notation ("describe" is the definition of a test suite, "it" is the definition of a test within any test suite, "expect" is the expectations that are checked in the test), Angular decided to use the existing experience and made a similar approach to tests. Angular comes with a testing library that includes wrappers for Jasmine's describe(), it(), and xit() functions, as well as adding functions such as beforeEach(), async(), fakeAsync(), and more.
The application is not configured or loaded during the test cycle. Therefore, Angular provides a helper class named TestBed, which allows you to declare modules, components, providers, etc. This class includes functions such as configureTestingModule(), createComponent(), inject (), and more. Here is an example from the standard Angular application on how to create a test suite.
File: test.ts
// This file is required by karma.conf.js and loads recursively all the .spec and framework files
import 'zone.js/testing';
import { getTestBed } from '@angular/core/testing';
import {
BrowserDynamicTestingModule,
platformBrowserDynamicTesting
} from '@angular/platform-browser-dynamic/testing';
declare const require: {
context(path: string, deep?: boolean, filter?: RegExp): {
keys(): string[];
<T>(id: string): T;
};
};
// First, initialize the Angular testing environment.
getTestBed().initTestEnvironment(
BrowserDynamicTestingModule,
platformBrowserDynamicTesting()
);
// Then we find all the tests.
const context = require.context('./', true, /\.spec\.ts$/);
// And load the modules.
context.keys().map(context);
Documenting projects on Angular
Documentation tools are available for the Angular application and related infrastructure:
- Compodoc: A great tool for creating documentation for your application.
- AngularDoc: Website of "Angular Application Architecture and Rendering".
- NgModule-Viz: Visualizing Relationships Between NgModules and Dependencies in Angular.
- Storybook for Angular: A perfect tool for user interface development. It uses the concept of isolating components.
- Angular DevTools: this is a great Chrome extension for debugging and profiling.
An example of what the documentation looks like in Compdoc:
Image from: https://github.com/compodoc/compodoc
Conclusion
In conclusion of this article, we’d like to highlight that Angular is a powerful and dynamically developing platform for writing modern front-end for web applications. This platform has all the modern components and integrates with Material Design and other component sets. Simultaneously, it is relatively convenient for front-end and full-stack developers to learn and master.
Svitla Systems has extensive experience in the development of front-end portions of software projects for our customers. We also have a large arsenal of solutions for Angular. Our front-end and full-stack specialists provide a quality solution that meets modern UI/UX requirements. You can contact Svitla Systems to assist with both existing projects and with projects at the initial cycle stage.
In the future, systems like Angular will be more and more advanced and convenient for both developers and end-users. The prospects for developing a front-end for your future project on Angular at the moment are not limited to anything, and thousands of modern web systems have been written using this wonderful platform.
Let's discuss your project
We look forward to learning more and consulting you about your product idea or helping you find the right solution for an existing project.
Your message is received. Svitla's sales manager of your region will contact you to discuss how we could be helpful.