Node.js is an open-source platform for executing high-performance web applications written in JavaScript. The founder of the platform is Ryan Dahl. Previously, JavaScript was used to process data in a browser to third parties, then Node.js provided the ability to execute JavaScript scripts on the server and send the result to the user. The Node.js platform has turned JavaScript into a server-side language with a large developer community.
You can read this blog about the success of well-known companies that use Node.js in production: Netflix, PayPal, Trello, LinkedIn, Yahoo, Uber, Groupon, eBay, Wallmart, and many others.
History
Quite a long time ago, attempts were made to implement JavaScript on the server-side to maintain a unified technology and programming language on the client-side and the server-side. In 1996, Netscape made attempts to create server-side JavaScript (Server-side JavaScript - SSJS). However, for some reason, the technology was not widely adopted.
Node.js was written initially by Ryan Dahl in 2009 and presented at the European JSConf on November 8, 2009. Now Node.js Foundation maintains and develops future releases by a wide and stable community. Here you can find a list of other JavaScript implementations but it seems Node.js is most widely known.
Various Server-Side Implementations
For example, you have your super-duper project, or you are planning to turn the world upside down with the help of your new web solution. What can you choose in terms of programming language and server-side runtime? There are a lot of choices, but how effective are they and how much will you be able to adapt all these miracles in the future
PHP
PHP is a scripting programming language that was created to generate HTML pages on the webserver side. PHP is one of the most common languages used in web development. To implement large and long-term projects, a modern PHP developer needs to take care of the code architecture, apply design patterns, write code in accordance with the principles of the chosen architecture and maintain high code coverage of the unit tests. But the area of PHP Backend development requires knowledge not only of the PHP language, but also knowledge of tools such as databases, queues, cache servers, without which a modern web application is unthinkable. This language and the corresponding backend work well, but there are also many complaints, such as those described in this article.
Ruby or rather Ruby-on-Rails
Ruby on Rails (RoR or Rails) is a multi-level MVC framework for building web applications using relational and NoSQL databases. The framework is written in the Ruby programming language. Rails is suitable both for the development of ordinary sites that should be really fast, fault-tolerant and work under high load, as well as for web applications with complex business logic and dynamic web interfaces. Ruby on Rails is open source software and is distributed under the MIT license. The visible drawback of this framework is a very difficult entry barrier since you won’t be able to study its peculiar syntax and programming approaches.
Python
With Python, we were all a little luckier. Firstly, the entry threshold is not very high; the Python programming language is easy to learn if you are not bothered by the absence of brackets at the beginning and at the end of the executable block since spaces are used instead. Secondly, there are many good frameworks, starting with the Flask microframework and ending with the full-fledged Django framework. With the speed of execution on the backend, everything is fine there too.
Java
But with Java, it’s not so simple. Firstly, you need to find enough highly qualified specialists in Java, and secondly, you need to correctly use the framework for the backend, for example, Spring. This will probably be one of the fastest decisions in terms of implementation on the backend, but experience shows that the time of project development and maintenance efforts are a concern.
If you have not seen your programming language or platform (C # .NET or Go or any other language or backend engine) in this list, we want to assure you that there are quite a large number of options and each of them individually deserves respect and has its advantages over other solutions.
Node.js advantages
But the advantage of Node.js can be described unambiguously and with one phrase - you have in your project one and the same programming language on the backend and frontend, and that is... Javascript!
And it is simply invaluable when you have Javascript on both sides, you are in the same development environment and you can use the code repeatedly on the backend and frontend. Saves time, budget and nerves with the certainty of support for the project for the future. In addition, Javascript is a language that has been used for more than 20 years, and Node.js is a backend that has been used for more than 10 years. This technology is already well established in the market.
Fig. 1. Node.js structure and layers
The next advantage of Node.JS is open-source with MIT license. MIT License is a group of licenses developed by the Massachusetts Institute of Technology for the distribution of free software.
Pros are the following:
- licenses are not a “copyleft”
- since there is no copyright for this license, other groups have the right to use and modify it to meet their goals
- clearly speaks of the rights of the end-user, including the rights to use, copy, modify, incorporate into another source code, publish, distribute, sublicense and/or sell licensed software
- the license is considered an academic license, that is, recognized as fit for use in the field of scientific development
Cons of this license are:
- MIT license is most consistent with the three-point BSD License.
In general, this is a very good license to use in any kind of project.
Another advantage is the use of the Google V8 engine, which effectively executes Javascript code. This actually ensured the success of the entire project of Node.JS. The engine code is open, Google supports it and the speed of execution of the source code is very fast. Plus, the same engine on the server-side and on most client platforms adds compatibility and ensures that the code will be executed the same way.
Single thread async computation model- first of all this model is simple to understand and at the same time very effective. Because of non-blocking input-output, it allows managing all tasks in a non-freezing mode in a single thread. If you really need multithreading then you can use a module like node-web worker-threads. If you follow simple rules of asynchronous programming, for example, do not use large cycles to process data, use events - then you can write clear and efficient code.
CommonJS (a voluntary working group) designs, prototypes, and standardizes various JavaScript APIs. They have ratified the standards for modules and packages - CommonJS defines a simple API for writing modules that can be used in the browser using the <script> tag, both with synchronous and with asynchronous loading. Structurally, the CommonJS module is a piece of JavaScript code ready for reuse that exports special objects that are available for use in any dependent code. CommonJS is increasingly being used as a standard format for JavaScript modules.
Unified API. Node.js can be combined with a database that supports JSON data (for example, MongoDB or CouchDB) and JSON for a unified JavaScript development stack and through the use of server-side development patterns (say MVC) Node.js allows you to reuse the same model and service interface between client and server sides.
Fig. 2. Typical structure of Node.js application.
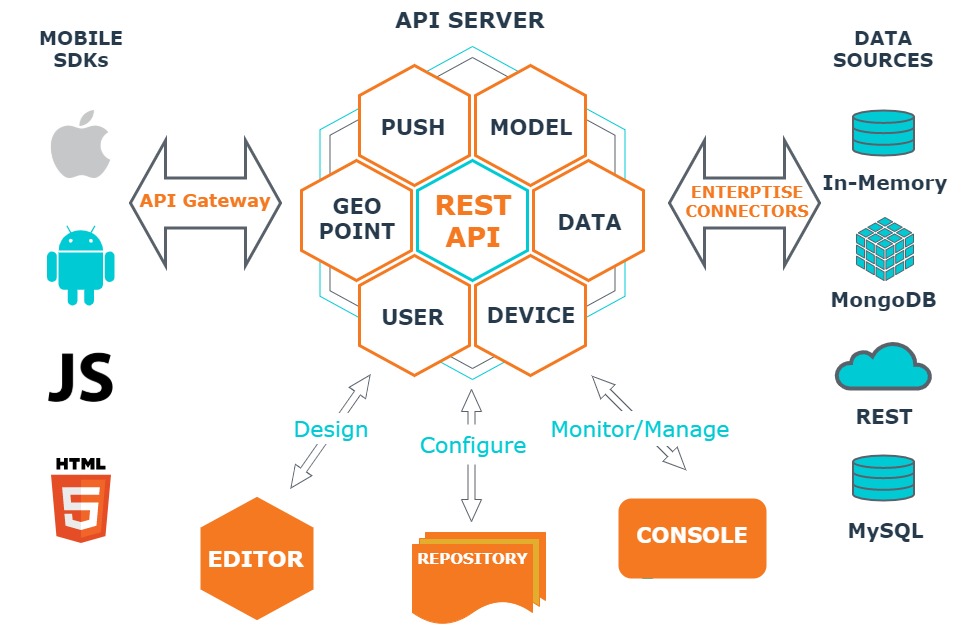
Object-oriented programming with Node.js
JavaScript is an object-oriented language, but the prototyping used in the language causes differences in working with objects compared to traditional class-oriented languages. In addition, JavaScript has a number of properties inherent in functional languages - functions as first-class objects, objects as lists, currying, anonymous functions, closures - which give the language additional flexibility.
The advantages of JavaScript compared to C++ are the following:
- objects with the possibility of introspection
- functions as objects of the first class
- automatic casting
- automatic garbage collection
- anonymous functions
Some developers welcome the use of OOP in Node.js, some completely deny it - there is no single answer. It all depends on the specifics of the project, the chosen architecture for using Node.js and the framework you are working on.
If you decide to use OOP in your project on Node.js then read this great article which gives an example of a carefully thought out use of OOP. In addition, follow the instructions in your framework for using OOP methods.
Modules and Frameworks
Node.js is a great system and one of the advantages is modules. For instance, you can use different modules and assemble the necessary configuration for your project. The most popular modules for Node.js are FileSystem, PDFKit, Cheerio, node-csv, Nodemailer and many others. You will always find a module that suits your needs and install it with the y npm package manager.
If you are developing a project on Node.js then you will definitely need some kind of framework. There is no point now in writing your own framework from scratch when you can use the most suitable ready-made one with an open license.
Express.js a web application framework for Node.js implemented as free and open-source software under the MIT license. This framework is designed to build web applications and APIs. Almost a standard framework for Node.js., Express is minimalistic and includes a large number of plug-ins.
Mojito is a Model-View-Controller (MVC) web application framework. It supports agile development, i.e. has built-in support for unit testing, supports internationalization, has syntax and coding convention check. Its architecture consists of server and client runtime which makes a very good environment for backend and frontend development. Also, Mojito has a system called Mojits, which supports the modules of a Mojito application.
Meteor or MeteorJS is a free and open web framework that uses Node.js. Meteor allows you to quickly create cross-platform applications (web, Android, iOS). It integrates with MongoDB and uses a distributed date protocol and post-subscription design template to automatically update data on the client without having to write the appropriate code for synchronization. To communicate with modern browsers, the Distributed Data Protocol (DDP) is used, supported by WebSockets. If there is no support for WebSockets and DDP, then Meteor uses AJAX.
Socket.IO is a JavaScript library for web applications and real-time data exchange. It consists of two parts: a client that runs in the browser and a server for Node.js. Both components have a similar application interface. Like Node.js, Socket.IO is event-oriented. Socket.IO can be used as a shell for WebSocket. It contains many other functions, including broadcasting to several sockets, storing data associated with each client, and asynchronous input/output.
If you need more information about frameworks for Node.js please refer to this great article.
Deploying Node.js to Cloud
The main cloud vendors found Node.js to be a cool platform and quickly deployed support for this environment. As in any cloud system, we get a ready-made infrastructure, the ability to scale, we pay only for the services that we consume, and we can transfer the system to different geolocations. All the goodies of cloud computing will be inherent in projects on Node.js in the cloud.
So who is supporting Node.js in the cloud now? Here is the list, which we hope will convince you completely:
- Amazon Cloud
- Microsoft Azure
- Digital Ocean
- Heroku
- Google Cloud
- Redhat OpenShift
There are other cloud providers that can support Node.js, but here we can’t list them all. You can check with your cloud provider to see how Node.js is supported and easily run your project in the desired cloud system.
Testing for Node.js
Of course, modern development, including Node.js, cannot work effectively without automated testing. You may like or dislike test-driven development, but definitely, understand the importance of testing in small and large projects.
During the development process, we check from time to time whether the function works correctly. The easiest way to check is to run it, for example in the console, and see the result.
If something is wrong, fix it, run it again - see the result ... and so on until the code is right. But such manual launches are a very imperfect means of verification.
A simple approach for testing is using Node.js’ assert module. The assert module provides a simple set of assertion statements that you can use to validate conditions. The module is designed for internal use by Node.js but can be used in application code using require ('assert'). However, assert is not a testing environment and cannot be used as a general-purpose claims library.
It is highly recommended to use Mocha for testing. Mocha is a library that contains common functions for testing, including describe and it. And Chai is a library that supports a variety of functions for checks. There are different “styles” for checking results but most often assert.equal is used. Sinon is designed for emulation and tricky substitution of functions with “stubs”.
Conclusion
What can be said about choosing a platform for the backend of the project in the modern version? Of course, the choice of technology is yours, but honestly, most of the arguments speak in favor of Node.js. This applies both for a small home project that you need to quickly build in a couple of hours, and a huge highly loaded service at an enterprise level.
The advantages of using JavaScript on the backend and frontend at the same time will clearly outweigh almost any other system (well, almost).
Node.js technology is already well-established, proven by powerful companies in business, it will be easy for you to find qualified developers and you will be able to select the correct necessary framework for development.
It remains only to wish you the successful use of Node.js in your projects.